public void takePhoto()
{
//Intent to launch camara
Intent intent = new Intent(MediaStore.ACTION_IMAGE_CAPTURE);
//Uri creattion to strore images
mImageCaptureUri = Uri.fromFile(new File(Environment.getExternalStorageDirectory()+ "/" + String.valueOf(System.currentTimeMillis())+ ".jpg"));
intent.putExtra(android.provider.MediaStore.EXTRA_OUTPUT, mImageCaptureUri);
startActivityForResult(intent, 1);
}
protected void onActivityResult(int requestCode, int resultCode, Intent imageReturnedIntent)
{
super.onActivityResult(requestCode, resultCode, imageReturnedIntent);
if(requestCode == 1)
{
if(resultCode == RESULT_OK)
{
try
{
ivPhoto.setBackgroundResource(0);
ivPhoto.setImageBitmap(BitmapFactory.decodeStream(new FileInputStream(new File(mImageCaptureUri.getPath()))));
}
catch (FileNotFoundException e)
{
e.printStackTrace();
}
}
else if(resultCode == RESULT_CANCELED)
{
Log.d("Result: ", "Launch Cancelled.");
}
}
}
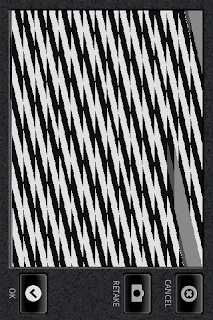
{
//Intent to launch camara
Intent intent = new Intent(MediaStore.ACTION_IMAGE_CAPTURE);
//Uri creattion to strore images
mImageCaptureUri = Uri.fromFile(new File(Environment.getExternalStorageDirectory()+ "/" + String.valueOf(System.currentTimeMillis())+ ".jpg"));
intent.putExtra(android.provider.MediaStore.EXTRA_OUTPUT, mImageCaptureUri);
startActivityForResult(intent, 1);
}
protected void onActivityResult(int requestCode, int resultCode, Intent imageReturnedIntent)
{
super.onActivityResult(requestCode, resultCode, imageReturnedIntent);
if(requestCode == 1)
{
if(resultCode == RESULT_OK)
{
try
{
ivPhoto.setBackgroundResource(0);
ivPhoto.setImageBitmap(BitmapFactory.decodeStream(new FileInputStream(new File(mImageCaptureUri.getPath()))));
}
catch (FileNotFoundException e)
{
e.printStackTrace();
}
}
else if(resultCode == RESULT_CANCELED)
{
Log.d("Result: ", "Launch Cancelled.");
}
}
}
Call takePhoto() in the button click.
Note:
In the manifast.xml we have to add permissions.android.permission.WRITE_EXTERNAL_STORAGE
android.hardware.camera
Output:
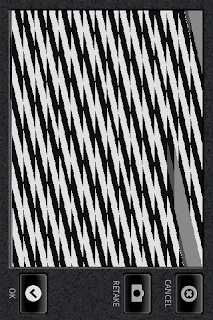