Using Build Class we will get the details of the mobile like device name, device info...
Build . MODEL ---- The visible device name.
Build . PRODUCT --- The name of the overall product.
Build . MANUFACTURER -- The manufacturer of the product.
For More Details about this class go through the link.
http://developer.android.com/reference/android/os/Build.html
Android Coding Examples - Android Code Samples
Sample Android codes, Android development tips, Android coding examples
July 09, 2011
July 08, 2011
Code Sample for Downlaoding Image via Internet in Android
public Bitmap downloadImageFromWeb(String url)
{
Bitmap bitmap = null;
InputStream in;
try
{
//Creation of url
URL url_img = new URL(url);
//Establishing Connection
in = url_img.openStream();
//Converting input stream to bitmap
bitmap = BitmapFactory.decodeStream(in);
//Closing the input stream
in.close();
}
catch (MalformedURLException e)
{
e.printStackTrace();
}
catch (IOException e)
{
e.printStackTrace();
}
return bitmap;
}
{
Bitmap bitmap = null;
InputStream in;
try
{
//Creation of url
URL url_img = new URL(url);
//Establishing Connection
in = url_img.openStream();
//Converting input stream to bitmap
bitmap = BitmapFactory.decodeStream(in);
//Closing the input stream
in.close();
}
catch (MalformedURLException e)
{
e.printStackTrace();
}
catch (IOException e)
{
e.printStackTrace();
}
return bitmap;
}
July 06, 2011
Android Code Sample for XML DomParsing using HashMap
You can use the sample given below for testing how to parse a xml using DomParsing and can get a clear idea to use it on your work.
parse.xml :
<Books>
<Book>
<Name>Cryptography</Name>
<Author>Harish</Author>
<Price>$200</Price>
</Book>
<Book>
<Name>Android</Name>
<Author>Harish</Author>
<Price>$250</Price>
</Book>
</Books>
Method to parse xml using dom :
public HashMap doDomParsing(String strUrl, String strParent, String[] child)
{
HashMap hmap, hmapchild = null;
URL url;
hmap = new HashMap();
try
{
//url = new URL(strUrl);
DocumentBuilderFactory dbf = DocumentBuilderFactory.newInstance();
DocumentBuilder db = dbf.newDocumentBuilder();
NodeList nodeList = doc.getElementsByTagName("item");
for (int i = 0; i < nodeList.getLength(); i++)
{
Node node = nodeList.item(i);
NodeList properties = node.getChildNodes();
hmapchild = new HashMap();
for(int j=0 ; j < properties.getLength() ; j++)
{
Node property = properties.item(j);
String nodename = property.getNodeName();
Log.e("Node NAME", nodename);
for(int inew =0 ;inew < child.length ; inew++)
{
if(nodename.equalsIgnoreCase(child[inew]))
{
hmapchild.put(nodename, property.getFirstChild().getNodeValue());
}
}
}
hmap.put(i, hmapchild);
}
}
catch (Exception e)
{
// TODO Auto-generated catch block
e.printStackTrace();
}
return hmap;
}
For this method we have to pass three parameters.
URL
PARENT NODE
CHILDREN
For the above xml we are parsing local file presented at raw folder. so url is empty.
Parent is Book
child array is {Name, Author, Price}
That's it. It will parse the data store the values in Hash Map and returns that Hash Map.
parse.xml :
<Books>
<Book>
<Name>Cryptography</Name>
<Author>Harish</Author>
<Price>$200</Price>
</Book>
<Book>
<Name>Android</Name>
<Author>Harish</Author>
<Price>$250</Price>
</Book>
</Books>
Method to parse xml using dom :
public HashMap doDomParsing(String strUrl, String strParent, String[] child)
{
HashMap hmap, hmapchild = null;
URL url;
hmap = new HashMap();
try
{
//url = new URL(strUrl);
DocumentBuilderFactory dbf = DocumentBuilderFactory.newInstance();
DocumentBuilder db = dbf.newDocumentBuilder();
Document doc = db.parse(new InputSource(getClass().getResourceAsStream("/res/raw/parse.xml")));
NodeList nodeList = doc.getElementsByTagName("item");
for (int i = 0; i < nodeList.getLength(); i++)
{
Node node = nodeList.item(i);
NodeList properties = node.getChildNodes();
hmapchild = new HashMap();
for(int j=0 ; j < properties.getLength() ; j++)
{
Node property = properties.item(j);
String nodename = property.getNodeName();
Log.e("Node NAME", nodename);
for(int inew =0 ;inew < child.length ; inew++)
{
if(nodename.equalsIgnoreCase(child[inew]))
{
hmapchild.put(nodename, property.getFirstChild().getNodeValue());
}
}
}
hmap.put(i, hmapchild);
}
}
catch (Exception e)
{
// TODO Auto-generated catch block
e.printStackTrace();
}
return hmap;
}
For this method we have to pass three parameters.
URL
PARENT NODE
CHILDREN
For the above xml we are parsing local file presented at raw folder. so url is empty.
Parent is Book
child array is {Name, Author, Price}
That's it. It will parse the data store the values in Hash Map and returns that Hash Map.
How to Automatically Start an Android Service after Reboot
Always a service is started by us manually that means it was not start by default. If we switch off the mobile then all the running services will stop.
For example if we are running a service. If we reboot the mobile then all the running services are stopped. So we have to run the service again.
By default android broadcasts a intent (android.intent.action.BOOT_COMPLETED)at the time of booting. So we have to receive that and in that we again start a service.
This should be accomplished by using BroadCast Receiver.
import android.content.BroadcastReceiver;
import android.content.Context;
import android.content.Intent;
import android.util.Log;
public class BootBroadcastReceiver extends BroadcastReceiver
{
public void onReceive(Context context, Intent intent)
{
Log.i("Receiver Called.", "start the service.");
}
}
We have to add this receiver in the manifast.xml. The code is as follows.
<!-- Receiver -->
<receiver android:name=".BootBroadcastReceiver">
<intent-filter>
<action android:name="android.intent.action.BOOT_COMPLETED" />
<category android:name="android.intent.category.HOME" />
</intent-filter>
</receiver>
For example if we are running a service. If we reboot the mobile then all the running services are stopped. So we have to run the service again.
By default android broadcasts a intent (android.intent.action.BOOT_COMPLETED)at the time of booting. So we have to receive that and in that we again start a service.
This should be accomplished by using BroadCast Receiver.
import android.content.BroadcastReceiver;
import android.content.Context;
import android.content.Intent;
import android.util.Log;
public class BootBroadcastReceiver extends BroadcastReceiver
{
public void onReceive(Context context, Intent intent)
{
Log.i("Receiver Called.", "start the service.");
}
}
We have to add this receiver in the manifast.xml. The code is as follows.
<!-- Receiver -->
<receiver android:name=".BootBroadcastReceiver">
<intent-filter>
<action android:name="android.intent.action.BOOT_COMPLETED" />
<category android:name="android.intent.category.HOME" />
</intent-filter>
</receiver>
July 02, 2011
How to Get Current Location of User in Android
In Android Three Approaches are preferred to Get Current Location
-> Using GPS
-> Cell - Id
-> Wi-Fi
The above three gives a clue to get the current location. Gps gives the results accurately. But it consumes Battery. Android Network Location Provider get the current location based wifi signals and cell tower. We have to use both or a single to Get the Current Location
LocationManager myManager;
MyLocListener loc;
loc = new MyLocListener();
myManager = (LocationManager) context.getSystemService(Context.LOCATION_SERVICE);
myManager.requestLocationUpdates(LocationManager.GPS_PROVIDER, 0, 0, loc );
package com.gps.activities;
import android.location.Location;
import android.location.LocationListener;
import android.os.Bundle;
public class MyLocListener implements LocationListener{
//Here you will get the latitude and longitude of a current location.
@Override
public void onLocationChanged(Location location)
{
if(location != null)
{
Log.e("Latitude :", ""+location.getLatitude());
Log.e("Latitude :", ""+location.getLongitude());
}}
@Override
public void onProviderDisabled(String provider) {
// TODO Auto-generated method stub
}
@Override
public void onProviderEnabled(String provider) {
// TODO Auto-generated method stub
}
@Override
public void onStatusChanged(String provider, int status, Bundle extras) {
// TODO Auto-generated method stub
}
}
Note: Include the below permissions in manifast.xml to listen Location Updates.
<uses-permission android:name="android.permission.ACCESS_FINE_LOCATION" />
<uses-permission android:name="android.permission.ACCESS_COARSE_LOCATION"/>Note : If you want more details about Obtaining User Location/ Get User Location
refer the below link. http://developer.android.com/guide/topics/location/obtaining-user-location.html
-> Using GPS
-> Cell - Id
-> Wi-Fi
The above three gives a clue to get the current location. Gps gives the results accurately. But it consumes Battery. Android Network Location Provider get the current location based wifi signals and cell tower. We have to use both or a single to Get the Current Location
LocationManager myManager;
MyLocListener loc;
loc = new MyLocListener();
myManager = (LocationManager) context.getSystemService(Context.LOCATION_SERVICE);
myManager.requestLocationUpdates(LocationManager.GPS_PROVIDER, 0, 0, loc );
package com.gps.activities;
import android.location.Location;
import android.location.LocationListener;
import android.os.Bundle;
public class MyLocListener implements LocationListener{
//Here you will get the latitude and longitude of a current location.
@Override
public void onLocationChanged(Location location)
{
if(location != null)
{
Log.e("Latitude :", ""+location.getLatitude());
Log.e("Latitude :", ""+location.getLongitude());
}}
@Override
public void onProviderDisabled(String provider) {
// TODO Auto-generated method stub
}
@Override
public void onProviderEnabled(String provider) {
// TODO Auto-generated method stub
}
@Override
public void onStatusChanged(String provider, int status, Bundle extras) {
// TODO Auto-generated method stub
}
}
Note: Include the below permissions in manifast.xml to listen Location Updates.
<uses-permission android:name="android.permission.ACCESS_FINE_LOCATION" />
<uses-permission android:name="android.permission.ACCESS_COARSE_LOCATION"/>Note : If you want more details about Obtaining User Location/ Get User Location
refer the below link. http://developer.android.com/guide/topics/location/obtaining-user-location.html
June 28, 2011
How to Attach Images after Launching Camera in Android
public void takePhoto()
{
//Intent to launch camara
Intent intent = new Intent(MediaStore.ACTION_IMAGE_CAPTURE);
//Uri creattion to strore images
mImageCaptureUri = Uri.fromFile(new File(Environment.getExternalStorageDirectory()+ "/" + String.valueOf(System.currentTimeMillis())+ ".jpg"));
intent.putExtra(android.provider.MediaStore.EXTRA_OUTPUT, mImageCaptureUri);
startActivityForResult(intent, 1);
}
protected void onActivityResult(int requestCode, int resultCode, Intent imageReturnedIntent)
{
super.onActivityResult(requestCode, resultCode, imageReturnedIntent);
if(requestCode == 1)
{
if(resultCode == RESULT_OK)
{
try
{
ivPhoto.setBackgroundResource(0);
ivPhoto.setImageBitmap(BitmapFactory.decodeStream(new FileInputStream(new File(mImageCaptureUri.getPath()))));
}
catch (FileNotFoundException e)
{
e.printStackTrace();
}
}
else if(resultCode == RESULT_CANCELED)
{
Log.d("Result: ", "Launch Cancelled.");
}
}
}
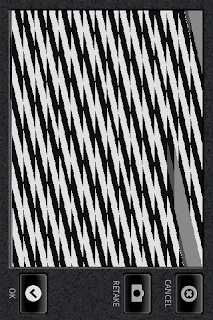
{
//Intent to launch camara
Intent intent = new Intent(MediaStore.ACTION_IMAGE_CAPTURE);
//Uri creattion to strore images
mImageCaptureUri = Uri.fromFile(new File(Environment.getExternalStorageDirectory()+ "/" + String.valueOf(System.currentTimeMillis())+ ".jpg"));
intent.putExtra(android.provider.MediaStore.EXTRA_OUTPUT, mImageCaptureUri);
startActivityForResult(intent, 1);
}
protected void onActivityResult(int requestCode, int resultCode, Intent imageReturnedIntent)
{
super.onActivityResult(requestCode, resultCode, imageReturnedIntent);
if(requestCode == 1)
{
if(resultCode == RESULT_OK)
{
try
{
ivPhoto.setBackgroundResource(0);
ivPhoto.setImageBitmap(BitmapFactory.decodeStream(new FileInputStream(new File(mImageCaptureUri.getPath()))));
}
catch (FileNotFoundException e)
{
e.printStackTrace();
}
}
else if(resultCode == RESULT_CANCELED)
{
Log.d("Result: ", "Launch Cancelled.");
}
}
}
Call takePhoto() in the button click.
Note:
In the manifast.xml we have to add permissions.android.permission.WRITE_EXTERNAL_STORAGE
android.hardware.camera
Output:
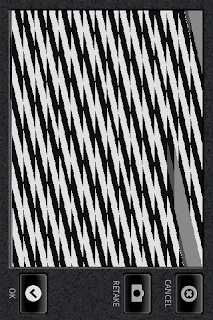
June 24, 2011
How to Remove Dull Background on Active Android Application
While running progress dialog the screen becomes dull. In order to avoid that blur the following code will helpful.
Here i am applying properties to our progress dialog. In this example "lp.dimAmount:" is the attribute to set the dim amount .
pdlg = ProgressDialog.show(getParent(), "", "Loading..");
//Setting the properties
WindowManager.LayoutParams lp = pdlg.getWindow().getAttributes();
lp.dimAmount=0.0f;
//Applying to the screen
pdlg.getWindow().setAttributes(lp);
Here i am applying properties to our progress dialog. In this example "lp.dimAmount:" is the attribute to set the dim amount .
pdlg = ProgressDialog.show(getParent(), "", "Loading..");
//Setting the properties
WindowManager.LayoutParams lp = pdlg.getWindow().getAttributes();
lp.dimAmount=0.0f;
//Applying to the screen
pdlg.getWindow().setAttributes(lp);
Subscribe to:
Posts (Atom)